Lab 02
Lab02: Java Comand Line and JGrasp
Compiling Java Lab 2A
Introduction
The goal for this lab is to gain experience editing, compiling and executing Java programs in the terminal and through the JGrasp IDE. You may work on this lab individually or in a group of no more than three people.
Compiling and Executing Java in the Terminal
Each of the steps below should be completed entirely inside the terminal: no GUI applications allowed. Refer back to the Unix Tutorial for Beginners if you need to refresh yourself on the necessary commands.
- Create a cs149 folder on your desktop
mkdir cs149 - Move into the cs149 directory:
cd cs149 - Create a folder inside your home directory named
lab02
.
mkdir lab02 - Move into the lab02 directory:
cd lab02 - Copy the file
Welcome.java
by doing a Right-Click Save-As and saving it to your lab02 folder. - Compile
Welcome.java
:$ javac Welcome.java
- If all goes well, this command should not produce any output to the terminal window, but it should create a new file named
Welcome.class
. - Examine the contents of
Welcome.class
using thecat
command.Don't worry! The contents shouldn't make sense to you. They wouldn't make much sense to anyone. Why not?
(Click for the answer.)
- Now that
Welcome.java
has been compiled, it can be executed:$ java Welcome
Notice that the.class
extension is not included. - Congratulations! You've successfully compiled and executed your first Java program.
Editing Files in the Terminal
Normally, we will be using an Integrated Development Environment (IDE) to edit and compile Java programs. However, it can sometimes be convenient to edit a file directly in the terminal. There are many terminal-based editors. Today we'll try nano
because it is easy to use for beginners.
- Open
Welcome.java
usingnano
:$ nano Welcome.java
You should see something like the following:
The two lines of text at the bottom show the set of actions available in the editor. The "^" symbol indicates the "Ctrl" key. For example, pressing Ctrl-O will "WriteOut" (save) any changes you have made to the file.
- Edit the file so that the welcome message says
"It's REALLY fun."
instead of"It's fun."
. Save your changes and exit. - Try executing your program again:
$ java Welcome
Does the output reflect your changes? Why not?
(Click for the answer.)
- Recompile then Execute your modified Java program:
javac Welcome.java
java Welcome
Submission
Nothing for this portion submission will be at the end of Part B
Acknowledgements This lab is based on a lab designed by Nathan Sprague.
JGrasp Lab2B
Objectives
-
Use an IDE (Integrated Development Environment).
-
Edit, save, compile, and run a simple Java program.
-
Recognize and correct syntax errors in a Java program.
Key Terms
- source file
- the Java program as written by the programmer
- class file
- the executable program produced by the compiler
- compile
- process of checking syntax and producing a class file
- syntax error
- mistake in the source code that prevents compilation
- logic error
- mistake in the program that causes incorrect behavior
- execute
- the process of running a program on a computer
Part 1: Java Development Cycle
jGRASP is a text editor designed to simplify the process of editing, compiling and executing Java programs.
-
Open jGRASP and click "File –> Open" from the menu and select your Welcome.java file from Lab 2A above.
-
Replace the // Simple Java Program. line in Welcome.java with the information below.
Change the@author
to your name and@version
to today's date. Pay attention to all spelling, punctuation, and indentation./** * Welcome. * * @author Alvin Chao * @version 1/18/2016 */ public class Welcome
{
public static void main(String[] args)
{
System.out.println("Welcome to CS149!");
System.out.println("It's really fun.");
}
} - Indentation doesn't matter to the Java compiler, but proper indentation makes code much easier for humans to read. Click the "Generate CSD" button
in the toolbar to indent the code and display a "Control Structure Diagram". Clicking the "Remove CSD" button
removes the diagram, but leaves the code properly indented. Try it out.
-
Compile your Java program by clicking the
button on the toolbar(This does the same thing as javac from the terminal in Lab 2A.
-
If it compiles successfully you should see a message like the following under the "Compile Messages" tab:
----jGRASP exec: javac -g Welcome.java ----jGRASP: operation complete.
If you have other messages indicating errors, check your typing carefully. Your error message will give you the line number of the first place the compiler was "confused" by what you typed.
-
Upon successful compilation, examine the directory (use the file browser) in which you placed your Hello.java file and you should see a Hello.class file.
-
-
Execute your program from jGRASP by clicking the
button on the toolbar. Under the "Run I/O" tab, you should see:
----jGRASP exec: java Welcome Welcome to CS149!
If not, please see the instructor before proceeding.
It's really fun. ----jGRASP: operation complete.
You have just completed the "edit, save, compile, execute" cycle. Each time you change and save your program, you will need to recompile the source file to see the changes reflected in the executed program.
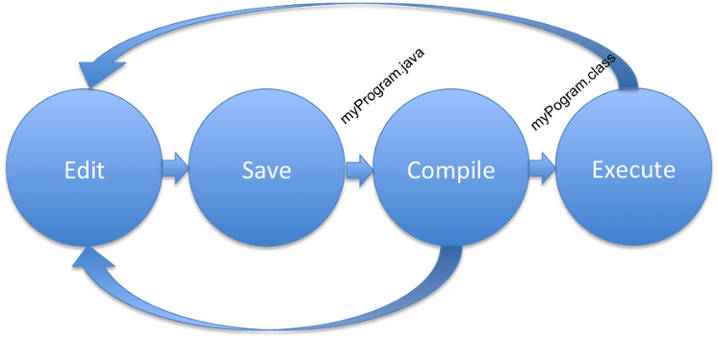
Part 2: Syntax Errors
Submission for Lab2
- Via canvas.jmu.edu by [Friday night 11:00pm] submit the Word of the Day and your Welcome.java file.
Extra work if You Have Time and want to get ahead...
For those who finish before the end of the lab period, I have an extra challenge for you. Write a program named Miles.java that converts miles to kilometers (i.e., 1 mile = 1.60934 km). You may use the example code in the Intro Java slides as a starting point.
Acknowledgments
This activity is based on a lab developed by Nathan Sprague based on a BlueJ lab originally developed by Chris Mayfield.